C++ if-else
In C++ programming, if statement is used to test the condition. There are various types of if statements in C++.
- if statement
- if-else statement
- nested if statement
- if-else-if ladder
C++ IF Statement
The C++ if statement tests the condition. It is executed if condition is true.
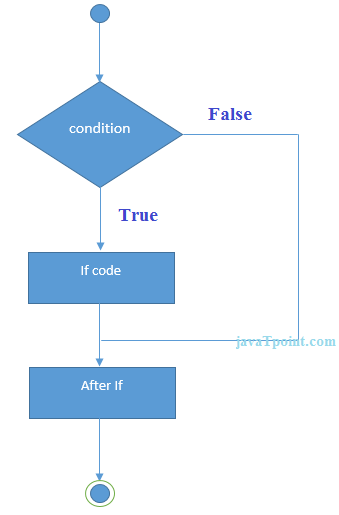
C++ If Example
Output:/p>
It is even number
C++ IF-else Statement
The C++ if-else statement also tests the condition. It executes if block if condition is true otherwise else block is executed.
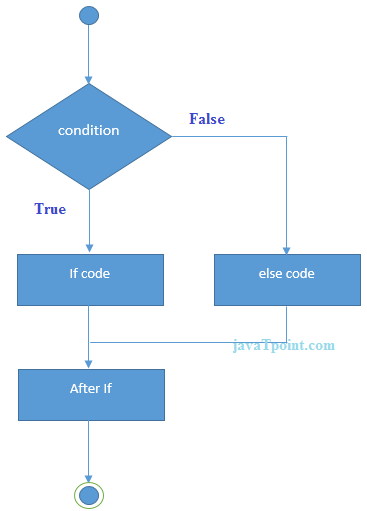
C++ If-else Example
Output:
It is odd number
C++ If-else Example: with input from user
Output:
Enter a number:11
It is odd number
Output:
Enter a number:12
It is even number
C++ IF-else-if ladder Statement
The C++ if-else-if ladder statement executes one condition from multiple statements.
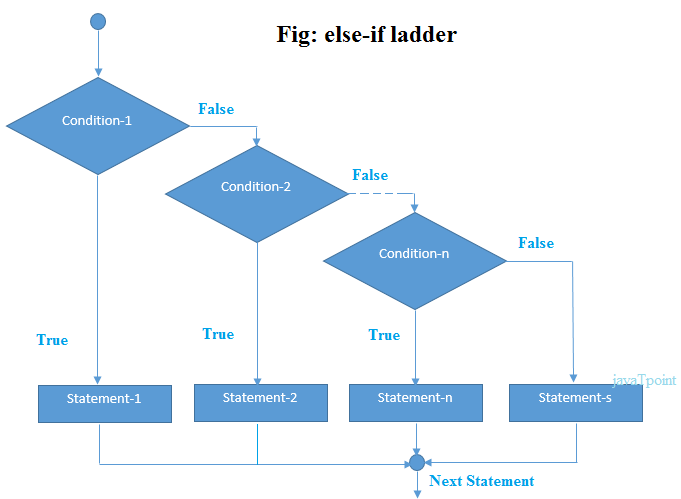
C++ If else-if Example
Output:
Enter a number to check grade:66
C Grade
Output:
Enter a number to check grade:-2
wrong number
C++ switch
The C++ switch statement executes one statement from multiple conditions. It is like if-else-if ladder statement in C++.
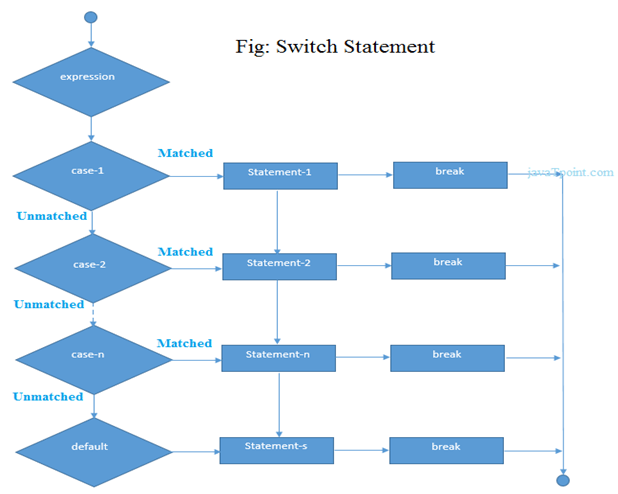
C++ Switch Example
Output:
Enter a number: 10 It is 10
Output:
Enter a number: 55 Not 10, 20 or 30
C++ For Loop
The C++ for loop is used to iterate a part of the program several times. If the number of iteration is fixed, it is recommended to use for loop than while or do-while loops.
The C++ for loop is same as C/C#. We can initialize variable, check condition and increment/decrement value.
Flowchart:
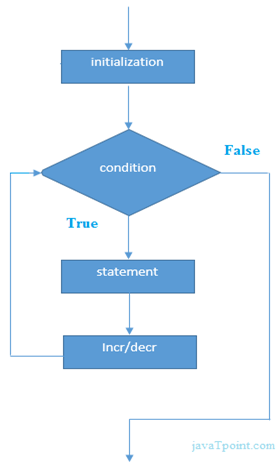
C++ For Loop Example
Output:
1 2 3 4 5 6 7 8 9 10
In C++, we can use for loop inside another for loop, it is known as nested for loop. The inner loop is executed fully when outer loop is executed one time. So if outer loop and inner loop are executed 4 times, inner loop will be executed 4 times for each outer loop i.e. total 16 times.
C++ Nested For Loop Example
Let's see a simple example of nested for loop in C++.
Output:
1 1 1 2 1 3 2 1 2 2 2 3 3 1 3 2 3 3
C++ Infinite For Loop
If we use double semicolon in for loop, it will be executed infinite times. Let's see a simple example of infinite for loop in C++.
Output:
Infinitive For Loop Infinitive For Loop Infinitive For Loop Infinitive For Loop Infinitive For Loop ctrl+c
C++ While loop
In C++, while loop is used to iterate a part of the program several times. If the number of iteration is not fixed, it is recommended to use while loop than for loop.
Flowchart:

C++ While Loop Example
Let's see a simple example of while loop to print table of 1.
Output:
1 2 3 4 5 6 7 8 9 10
C++ Nested While Loop Example
In C++, we can use while loop inside another while loop, it is known as nested while loop. The nested while loop is executed fully when outer loop is executed once.
Let's see a simple example of nested while loop in C++ programming language.
Output:
1 1 1 2 1 3 2 1 2 2 2 3 3 1 3 2 3 3
C++ Infinitive While Loop Example:
We can also create infinite while loop by passing true as the test condition.
Output:
Infinitive While Loop Infinitive While Loop Infinitive While Loop Infinitive While Loop Infinitive While Loop ctrl+c
C++ Do-While Loop
The C++ do-while loop is used to iterate a part of the program several times. If the number of iteration is not fixed and you must have to execute the loop at least once, it is recommended to use do-while loop.
The C++ do-while loop is executed at least once because condition is checked after loop body.
Flowchart:
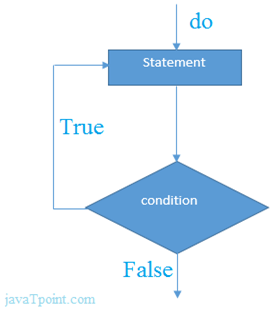
C++ do-while Loop Example
Let's see a simple example of C++ do-while loop to print the table of 1.
Output:
1 2 3 4 5 6 7 8 9 10
C++ Nested do-while Loop
In C++, if you use do-while loop inside another do-while loop, it is known as nested do-while loop. The nested do-while loop is executed fully for each outer do-while loop.
Let's see a simple example of nested do-while loop in C++.
Output:
1 1 1 2 1 3 2 1 2 2 2 3 3 1 3 2 3 3
C++ Infinitive do-while Loop
In C++, if you pass true in the do-while loop, it will be infinitive do-while loop.
C++ Infinitive do-while Loop Example
Output:
Infinitive do-while Loop Infinitive do-while Loop Infinitive do-while Loop Infinitive do-while Loop Infinitive do-while Loop ctrl+c
C++ Break Statement
The C++ break is used to break loop or switch statement. It breaks the current flow of the program at the given condition. In case of inner loop, it breaks only inner loop.
Flowchart:

C++ Break Statement Example
Let's see a simple example of C++ break statement which is used inside the loop.
Output:
1 2 3 4
C++ Break Statement with Inner Loop
The C++ break statement breaks inner loop only if you use break statement inside the inner loop.
Let's see the example code:
Output:
1 1 1 2 1 3 2 1 3 1 3 2 3 3
C++ Continue Statement
The C++ continue statement is used to continue loop. It continues the current flow of the program and skips the remaining code at specified condition. In case of inner loop, it continues only inner loop.
C++ Continue Statement Example
Output:
1 2 3 4 6 7 8 9 10
C++ Continue Statement with Inner Loop
C++ Continue Statement continues inner loop only if you use continue statement inside the inner loop.
Output:
1 1 1 2 1 3 2 1 2 3 3 1 3 2 3 3
C++ Goto Statement
The C++ goto statement is also known as jump statement. It is used to transfer control to the other part of the program. It unconditionally jumps to the specified label.
It can be used to transfer control from deeply nested loop or switch case label.
C++ Goto Statement Example
Let's see the simple example of goto statement in C++.
Output:
You are not eligible to vote! Enter your age: 16 You are not eligible to vote! Enter your age: 7 You are not eligible to vote! Enter your age: 22 You are eligible to vote!
Comments
Post a Comment