Life Cycle of a Servlet (Servlet Life Cycle)
Servlet class is loaded.The web container maintains the life cycle of a servlet instance. Let's see the life cycle of the servlet:
- Servlet instance is created.
- init method is invoked.
- service method is invoked.
- destroy method is invoked.
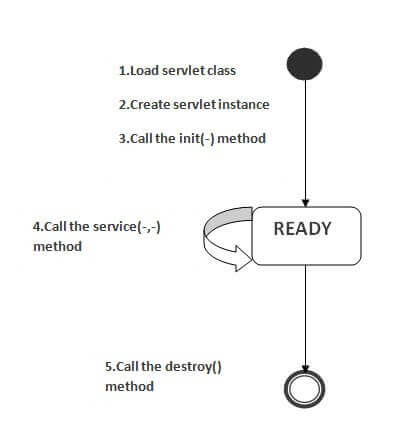
As displayed in the above diagram, there are three states of a servlet: new, ready and end. The servlet is in new state if servlet instance is created. After invoking the init() method, Servlet comes in the ready state. In the ready state, servlet performs all the tasks. When the web container invokes the destroy() method, it shifts to the end state.
1) Servlet class is loaded
The classloader is responsible to load the servlet class. The servlet class is loaded when the first request for the servlet is received by the web container.
2) Servlet instance is created
The web container creates the instance of a servlet after loading the servlet class. The servlet instance is created only once in the servlet life cycle.
3) init method is invoked
The web container calls the init method only once after creating the servlet instance. The init method is used to initialize the servlet. It is the life cycle method of the javax.servlet.Servlet interface. Syntax of the init method is given below: |
4) service method is invoked
The web container calls the service method each time when request for the servlet is received. If servlet is not initialized, it follows the first three steps as described above then calls the service method. If servlet is initialized, it calls the service method. Notice that servlet is initialized only once. The syntax of the service method of the Servlet interface is given below:
5) destroy method is invoked
The web container calls the destroy method before removing the servlet instance from the service. It gives the servlet an opportunity to clean up any resource for example memory, thread etc. The syntax of the destroy method of the Servlet interface is given below:
Steps to create a servlet example
The servlet example can be created by three ways:There are given 6 steps to create a servlet example. These steps are required for all the servers.
- By implementing Servlet interface,
- By inheriting GenericServlet class, (or)
- By inheriting HttpServlet class
The mostly used approach is by extending HttpServlet because it provides http request specific method such as doGet(), doPost(), doHead() etc.
Here, we are going to use apache tomcat server in this example. The steps are as follows:
- Create a directory structure
- Create a Servlet
- Compile the Servlet
- Create a deployment descriptor
- Start the server and deploy the project
- Access the servlet
1)Create a directory structures
The directory structure defines that where to put the different types of files so that web container may get the information and respond to the client.
The Sun Microsystem defines a unique standard to be followed by all the server vendors. Let's see the directory structure that must be followed to create the servlet.
As you can see that the servlet class file must be in the classes folder. The web.xml file must be under the WEB-INF folder.
2)Create a Servlet
There are three ways to create the servlet.
|
In this example we are going to create a servlet that extends the HttpServlet class. In this example, we are inheriting the HttpServlet class and providing the implementation of the doGet() method. Notice that get request is the default request. |
DemoServlet.java
3)Compile the servlet
For compiling the Servlet, jar file is required to be loaded. Different Servers provide different jar files:
Jar file | Server |
---|---|
1) servlet-api.jar | Apache Tomcat |
2) weblogic.jar | Weblogic |
3) javaee.jar | Glassfish |
4) javaee.jar | JBoss |
Two ways to load the jar file
- set classpath
- paste the jar file in JRE/lib/ext folder
Put the java file in any folder. After compiling the java file, paste the class file of servlet in WEB-INF/classes directory.
4)Create the deployment descriptor (web.xml file)
The deployment descriptor is an xml file, from which Web Container gets the information about the servet to be invoked.
The web container uses the Parser to get the information from the web.xml file. There are many xml parsers such as SAX, DOM and Pull.
There are many elements in the web.xml file. Here is given some necessary elements to run the simple servlet program.
web.xml file
Description of the elements of web.xml file
There are too many elements in the web.xml file. Here is the illustration of some elements that is used in the above web.xml file. The elements are as follows:
<web-app> represents the whole application. |
<servlet> is sub element of <web-app> and represents the servlet. |
<servlet-name> is sub element of <servlet> represents the name of the servlet. |
<servlet-class> is sub element of <servlet> represents the class of the servlet. |
<servlet-mapping> is sub element of <web-app>. It is used to map the servlet. |
<url-pattern> is sub element of <servlet-mapping>. This pattern is used at client side to invoke the servlet. |
5)Start the Server and deploy the project
To start Apache Tomcat server, double click on the startup.bat file under apache-tomcat/bin directory.
One Time Configuration for Apache Tomcat Server
You need to perform 2 tasks:
- set JAVA_HOME or JRE_HOME in environment variable (It is required to start server).
- Change the port number of tomcat (optional). It is required if another server is running on same port (8080).
1) How to set JAVA_HOME in environment variable?
To start Apache Tomcat server JAVA_HOME and JRE_HOME must be set in Environment variables.
Go to My Computer properties -> Click on advanced tab then environment variables -> Click on the new tab of user variable -> Write JAVA_HOME in variable name and paste the path of jdk folder in variable value -> ok -> ok -> ok.
Go to My Computer properties:
Click on advanced system settings tab then environment variables:
Click on the new tab of user variable or system variable:
Write JAVA_HOME in variable name and paste the path of jdk folder in variable value:
There must not be semicolon (;) at the end of the path.
After setting the JAVA_HOME double click on the startup.bat file in apache tomcat/bin. |
Note: There are two types of tomcat available:
|
It is the example of apache tomcat that needs to extract only. |
Now server is started successfully.
2) How to change port number of apache tomcat
Changing the port number is required if there is another server running on the same system with same port number.Suppose you have installed oracle, you need to change the port number of apache tomcat because both have the default port number 8080.
Open server.xml file in notepad. It is located inside the apache-tomcat/conf directory . Change the Connector port = 8080 and replace 8080 by any four digit number instead of 8080. Let us replace it by 9999 and save this file.
5) How to deploy the servlet project
Copy the project and paste it in the webapps folder under apache tomcat.
But there are several ways to deploy the project. They are as follows:
- By copying the context(project) folder into the webapps directory
- By copying the war folder into the webapps directory
- By selecting the folder path from the server
- By selecting the war file from the server
Here, we are using the first approach.
You can also create war file, and paste it inside the webapps directory. To do so, you need to use jar tool to create the war file. Go inside the project directory (before the WEB-INF), then write:
Creating war file has an advantage that moving the project from one location to another takes less time.
6) How to access the servlet
Open broser and write http://hostname:portno/contextroot/urlpatternofservlet. For example:
Comments
Post a Comment