Transaction Management in JDBC
Transaction represents a single unit of work.
The ACID properties describes the transaction management well. ACID stands for Atomicity, Consistency, isolation and durability.
Atomicity means either all successful or none.
Consistency ensures bringing the database from one consistent state to another consistent state.
Isolation ensures that transaction is isolated from other transaction.
Durability means once a transaction has been committed, it will remain so, even in the event of errors, power loss etc.
Advantage of Transaction Mangaement
fast performance It makes the performance fast because database is hit at the time of commit.
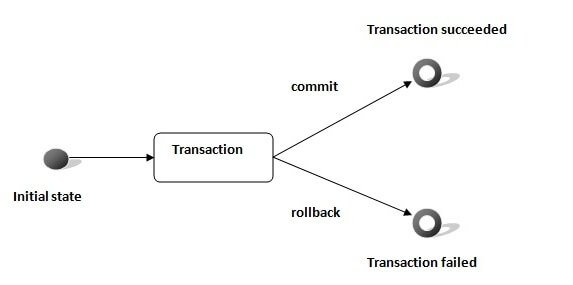
In JDBC, Connection interface provides methods to manage transaction.
Method | Description |
---|
void setAutoCommit(boolean status) | It is true bydefault means each transaction is committed bydefault. |
void commit() | commits the transaction. |
void rollback() | cancels the transaction. |
Simple example of transaction management in jdbc using Statement
Let's see the simple example of transaction management using Statement.
- import java.sql.*;
- class FetchRecords{
- public static void main(String args[])throws Exception{
- Class.forName("oracle.jdbc.driver.OracleDriver");
- Connection con=DriverManager.getConnection("jdbc:oracle:thin:@localhost:1521:xe","system","oracle");
- con.setAutoCommit(false);
-
- Statement stmt=con.createStatement();
- stmt.executeUpdate("insert into user420 values(190,'abhi',40000)");
- stmt.executeUpdate("insert into user420 values(191,'umesh',50000)");
-
- con.commit();
- con.close();
- }}
If you see the table emp400, you will see that 2 records has been added.
Example of transaction management in jdbc using PreparedStatement
Let's see the simple example of transaction management using PreparedStatement.
- import java.sql.*;
- import java.io.*;
- class TM{
- public static void main(String args[]){
- try{
-
- Class.forName("oracle.jdbc.driver.OracleDriver");
- Connection con=DriverManager.getConnection("jdbc:oracle:thin:@localhost:1521:xe","system","oracle");
- con.setAutoCommit(false);
-
- PreparedStatement ps=con.prepareStatement("insert into user420 values(?,?,?)");
-
- BufferedReader br=new BufferedReader(new InputStreamReader(System.in));
- while(true){
-
- System.out.println("enter id");
- String s1=br.readLine();
- int id=Integer.parseInt(s1);
-
- System.out.println("enter name");
- String name=br.readLine();
-
- System.out.println("enter salary");
- String s3=br.readLine();
- int salary=Integer.parseInt(s3);
-
- ps.setInt(1,id);
- ps.setString(2,name);
- ps.setInt(3,salary);
- ps.executeUpdate();
-
- System.out.println("commit/rollback");
- String answer=br.readLine();
- if(answer.equals("commit")){
- con.commit();
- }
- if(answer.equals("rollback")){
- con.rollback();
- }
-
-
- System.out.println("Want to add more records y/n");
- String ans=br.readLine();
- if(ans.equals("n")){
- break;
- }
-
- }
- con.commit();
- System.out.println("record successfully saved");
-
- con.close();
- }catch(Exception e){System.out.println(e);}
-
- }}
It will ask to add more records until you press n. If you press n, transaction is committed.
Batch Processing in JDBC
Instead of executing a single query, we can execute a batch (group) of queries. It makes the performance fast.
The java.sql.Statement and java.sql.PreparedStatement interfaces provide methods for batch processing.
Advantage of Batch Processing
Fast Performance
Methods of Statement interface
The required methods for batch processing are given below:
Method | Description |
---|
void addBatch(String query) | It adds query into batch. |
int[] executeBatch() | It executes the batch of queries. |
Example of batch processing in jdbc
Let's see the simple example of batch processing in jdbc. It follows following steps:
- Load the driver class
- Create Connection
- Create Statement
- Add query in the batch
- Execute Batch
- Close Connection
- import java.sql.*;
- class FetchRecords{
- public static void main(String args[])throws Exception{
- Class.forName("oracle.jdbc.driver.OracleDriver");
- Connection con=DriverManager.getConnection("jdbc:oracle:thin:@localhost:1521:xe","system","oracle");
- con.setAutoCommit(false);
-
- Statement stmt=con.createStatement();
- stmt.addBatch("insert into user420 values(190,'abhi',40000)");
- stmt.addBatch("insert into user420 values(191,'umesh',50000)");
-
- stmt.executeBatch();
-
- con.commit();
- con.close();
- }}
If you see the table user420, two records has been added.
Example of batch processing using PreparedStatement
- import java.sql.*;
- import java.io.*;
- class BP{
- public static void main(String args[]){
- try{
-
- Class.forName("oracle.jdbc.driver.OracleDriver");
- Connection con=DriverManager.getConnection("jdbc:oracle:thin:@localhost:1521:xe","system","oracle");
-
- PreparedStatement ps=con.prepareStatement("insert into user420 values(?,?,?)");
-
- BufferedReader br=new BufferedReader(new InputStreamReader(System.in));
- while(true){
-
- System.out.println("enter id");
- String s1=br.readLine();
- int id=Integer.parseInt(s1);
-
- System.out.println("enter name");
- String name=br.readLine();
-
- System.out.println("enter salary");
- String s3=br.readLine();
- int salary=Integer.parseInt(s3);
-
- ps.setInt(1,id);
- ps.setString(2,name);
- ps.setInt(3,salary);
-
- ps.addBatch();
- System.out.println("Want to add more records y/n");
- String ans=br.readLine();
- if(ans.equals("n")){
- break;
- }
-
- }
- ps.executeBatch();
-
- System.out.println("record successfully saved");
-
- con.close();
- }catch(Exception e){System.out.println(e);}
-
- }}
It will add the queries into the batch until user press n. Finally it executes the batch. Thus all the added queries will be fired.
JDBC RowSet
The instance of RowSet is the java bean component because it has properties and java bean notification mechanism. It is introduced since JDK 5.
It is the wrapper of ResultSet. It holds tabular data like ResultSet but it is easy and flexible to use.
The implementation classes of RowSet interface are as follows:
- JdbcRowSet
- CachedRowSet
- WebRowSet
- JoinRowSet
- FilteredRowSet
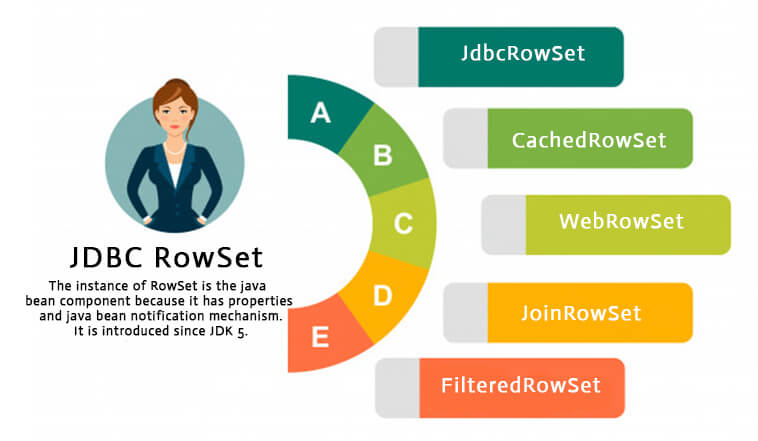
Let's see how to create and execute RowSet.
- JdbcRowSet rowSet = RowSetProvider.newFactory().createJdbcRowSet();
- rowSet.setUrl("jdbc:oracle:thin:@localhost:1521:xe");
- rowSet.setUsername("system");
- rowSet.setPassword("oracle");
-
- rowSet.setCommand("select * from emp400");
- rowSet.execute();
It is the new way to get the instance of JdbcRowSet since JDK 7.
Advantage of RowSet
The advantages of using RowSet are given below:
- It is easy and flexible to use
- It is Scrollable and Updatable bydefault
Simple example of JdbcRowSet
Let's see the simple example of JdbcRowSet without event handling code.
- import java.sql.Connection;
- import java.sql.DriverManager;
- import java.sql.ResultSet;
- import java.sql.Statement;
- import javax.sql.RowSetEvent;
- import javax.sql.RowSetListener;
- import javax.sql.rowset.JdbcRowSet;
- import javax.sql.rowset.RowSetProvider;
-
- public class RowSetExample {
- public static void main(String[] args) throws Exception {
- Class.forName("oracle.jdbc.driver.OracleDriver");
-
-
- JdbcRowSet rowSet = RowSetProvider.newFactory().createJdbcRowSet();
- rowSet.setUrl("jdbc:oracle:thin:@localhost:1521:xe");
- rowSet.setUsername("system");
- rowSet.setPassword("oracle");
-
- rowSet.setCommand("select * from emp400");
- rowSet.execute();
-
- while (rowSet.next()) {
-
- System.out.println("Id: " + rowSet.getString(1));
- System.out.println("Name: " + rowSet.getString(2));
- System.out.println("Salary: " + rowSet.getString(3));
- }
-
- }
- }
The output is given below:
Id: 55
Name: Om Bhim
Salary: 70000
Id: 190
Name: abhi
Salary: 40000
Id: 191
Name: umesh
Salary: 50000
Full example of Jdbc RowSet with event handling
To perform event handling with JdbcRowSet, you need to add the instance of RowSetListener in the addRowSetListener method of JdbcRowSet.
The RowSetListener interface provides 3 method that must be implemented. They are as follows:
1) public void cursorMoved(RowSetEvent event);
2) public void rowChanged(RowSetEvent event);
3) public void rowSetChanged(RowSetEvent event);
Let's write the code to retrieve the data and perform some additional tasks while cursor is moved, cursor is changed or rowset is changed. The event handling operation can't be performed using ResultSet so it is preferred now.
- import java.sql.Connection;
- import java.sql.DriverManager;
- import java.sql.ResultSet;
- import java.sql.Statement;
- import javax.sql.RowSetEvent;
- import javax.sql.RowSetListener;
- import javax.sql.rowset.JdbcRowSet;
- import javax.sql.rowset.RowSetProvider;
-
- public class RowSetExample {
- public static void main(String[] args) throws Exception {
- Class.forName("oracle.jdbc.driver.OracleDriver");
-
-
- JdbcRowSet rowSet = RowSetProvider.newFactory().createJdbcRowSet();
- rowSet.setUrl("jdbc:oracle:thin:@localhost:1521:xe");
- rowSet.setUsername("system");
- rowSet.setPassword("oracle");
-
- rowSet.setCommand("select * from emp400");
- rowSet.execute();
-
-
- rowSet.addRowSetListener(new MyListener());
-
- while (rowSet.next()) {
-
- System.out.println("Id: " + rowSet.getString(1));
- System.out.println("Name: " + rowSet.getString(2));
- System.out.println("Salary: " + rowSet.getString(3));
- }
-
- }
- }
-
- class MyListener implements RowSetListener {
- public void cursorMoved(RowSetEvent event) {
- System.out.println("Cursor Moved...");
- }
- public void rowChanged(RowSetEvent event) {
- System.out.println("Cursor Changed...");
- }
- public void rowSetChanged(RowSetEvent event) {
- System.out.println("RowSet changed...");
- }
- }
The output is as follows:
Cursor Moved...
Id: 55
Name: Om Bhim
Salary: 70000
Cursor Moved...
Id: 190
Name: abhi
Salary: 40000
Cursor Moved...
Id: 191
Name: umesh
Salary: 50000
Cursor Moved...
Anurag Rana
Educator CSE/IT
Comments
Post a Comment