Java JTable
The JTable class is used to display data in tabular form. It is composed of rows and columns.
JTable class declaration
Let's see the declaration for javax.swing.JTable class.
Commonly used Constructors:
Constructor | Description |
---|
JTable() | Creates a table with empty cells. |
JTable(Object[][] rows, Object[] columns) | Creates a table with the specified data. |
Java JTable Example
- import javax.swing.*;
- public class TableExample {
- JFrame f;
- TableExample(){
- f=new JFrame();
- String data[][]={ {"101","Amit","670000"},
- {"102","Jai","780000"},
- {"101","Sachin","700000"}};
- String column[]={"ID","NAME","SALARY"};
- JTable jt=new JTable(data,column);
- jt.setBounds(30,40,200,300);
- JScrollPane sp=new JScrollPane(jt);
- f.add(sp);
- f.setSize(300,400);
- f.setVisible(true);
- }
- public static void main(String[] args) {
- new TableExample();
- }
- }
Output:
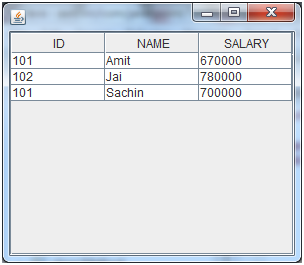
Java JTable Example with ListSelectionListener
- import javax.swing.*;
- import javax.swing.event.*;
- public class TableExample {
- public static void main(String[] a) {
- JFrame f = new JFrame("Table Example");
- String data[][]={ {"101","Amit","670000"},
- {"102","Jai","780000"},
- {"101","Sachin","700000"}};
- String column[]={"ID","NAME","SALARY"};
- final JTable jt=new JTable(data,column);
- jt.setCellSelectionEnabled(true);
- ListSelectionModel select= jt.getSelectionModel();
- select.setSelectionMode(ListSelectionModel.SINGLE_SELECTION);
- select.addListSelectionListener(new ListSelectionListener() {
- public void valueChanged(ListSelectionEvent e) {
- String Data = null;
- int[] row = jt.getSelectedRows();
- int[] columns = jt.getSelectedColumns();
- for (int i = 0; i < row.length; i++) {
- for (int j = 0; j < columns.length; j++) {
- Data = (String) jt.getValueAt(row[i], columns[j]);
- } }
- System.out.println("Table element selected is: " + Data);
- }
- });
- JScrollPane sp=new JScrollPane(jt);
- f.add(sp);
- f.setSize(300, 200);
- f.setVisible(true);
- }
- }
Output:
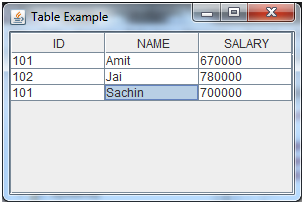
If you select an element in column NAME, name of the element will be displayed on the console:
- Table element selected is: Sachin
Java JList
The object of JList class represents a list of text items. The list of text items can be set up so that the user can choose either one item or multiple items. It inherits JComponent class.
JList class declaration
Let's see the declaration for javax.swing.JList class.
- public class JList extends JComponent implements Scrollable, Accessible
Commonly used Constructors:
Constructor | Description |
---|
JList() | Creates a JList with an empty, read-only, model. |
JList(ary[] listData) | Creates a JList that displays the elements in the specified array. |
JList(ListModel<ary> dataModel) | Creates a JList that displays elements from the specified, non-null, model. |
Commonly used Methods:
Methods | Description |
---|
Void addListSelectionListener(ListSelectionListener listener) | It is used to add a listener to the list, to be notified each time a change to the selection occurs. |
int getSelectedIndex() | It is used to return the smallest selected cell index. |
ListModel getModel() | It is used to return the data model that holds a list of items displayed by the JList component. |
void setListData(Object[] listData) | It is used to create a read-only ListModel from an array of objects. |
Java JList Example
- import javax.swing.*;
- public class ListExample
- {
- ListExample(){
- JFrame f= new JFrame();
- DefaultListModel<String> l1 = new DefaultListModel<>();
- l1.addElement("Item1");
- l1.addElement("Item2");
- l1.addElement("Item3");
- l1.addElement("Item4");
- JList<String> list = new JList<>(l1);
- list.setBounds(100,100, 75,75);
- f.add(list);
- f.setSize(400,400);
- f.setLayout(null);
- f.setVisible(true);
- }
- public static void main(String args[])
- {
- new ListExample();
- }}
Output:
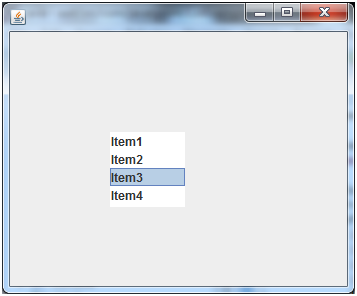
Java JList Example with ActionListener
- import javax.swing.*;
- import java.awt.event.*;
- public class ListExample
- {
- ListExample(){
- JFrame f= new JFrame();
- final JLabel label = new JLabel();
- label.setSize(500,100);
- JButton b=new JButton("Show");
- b.setBounds(200,150,80,30);
- final DefaultListModel<String> l1 = new DefaultListModel<>();
- l1.addElement("C");
- l1.addElement("C++");
- l1.addElement("Java");
- l1.addElement("PHP");
- final JList<String> list1 = new JList<>(l1);
- list1.setBounds(100,100, 75,75);
- DefaultListModel<String> l2 = new DefaultListModel<>();
- l2.addElement("Turbo C++");
- l2.addElement("Struts");
- l2.addElement("Spring");
- l2.addElement("YII");
- final JList<String> list2 = new JList<>(l2);
- list2.setBounds(100,200, 75,75);
- f.add(list1); f.add(list2); f.add(b); f.add(label);
- f.setSize(450,450);
- f.setLayout(null);
- f.setVisible(true);
- b.addActionListener(new ActionListener() {
- public void actionPerformed(ActionEvent e) {
- String data = "";
- if (list1.getSelectedIndex() != -1) {
- data = "Programming language Selected: " + list1.getSelectedValue();
- label.setText(data);
- }
- if(list2.getSelectedIndex() != -1){
- data += ", FrameWork Selected: ";
- for(Object frame :list2.getSelectedValues()){
- data += frame + " ";
- }
- }
- label.setText(data);
- }
- });
- }
- public static void main(String args[])
- {
- new ListExample();
- }}
Output:
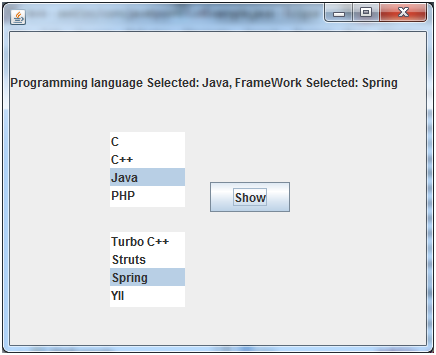
Java JOptionPane
The JOptionPane class is used to provide standard dialog boxes such as message dialog box, confirm dialog box and input dialog box. These dialog boxes are used to display information or get input from the user. The JOptionPane class inherits JComponent class.
JOptionPane class declaration
- public class JOptionPane extends JComponent implements Accessible
Common Constructors of JOptionPane class
Constructor | Description |
---|
JOptionPane() | It is used to create a JOptionPane with a test message. |
JOptionPane(Object message) | It is used to create an instance of JOptionPane to display a message. |
JOptionPane(Object message, int messageType | It is used to create an instance of JOptionPane to display a message with specified message type and default options. |
Common Methods of JOptionPane class
Methods | Description |
---|
JDialog createDialog(String title) | It is used to create and return a new parentless JDialog with the specified title. |
static void showMessageDialog(Component parentComponent, Object message) | It is used to create an information-message dialog titled "Message". |
static void showMessageDialog(Component parentComponent, Object message, String title, int messageType) | It is used to create a message dialog with given title and messageType. |
static int showConfirmDialog(Component parentComponent, Object message) | It is used to create a dialog with the options Yes, No and Cancel; with the title, Select an Option. |
static String showInputDialog(Component parentComponent, Object message) | It is used to show a question-message dialog requesting input from the user parented to parentComponent. |
void setInputValue(Object newValue) | It is used to set the input value that was selected or input by the user. |
Java JOptionPane Example: showMessageDialog()
- import javax.swing.*;
- public class OptionPaneExample {
- JFrame f;
- OptionPaneExample(){
- f=new JFrame();
- JOptionPane.showMessageDialog(f,"Hello, Welcome to Javatpoint.");
- }
- public static void main(String[] args) {
- new OptionPaneExample();
- }
- }
Output:
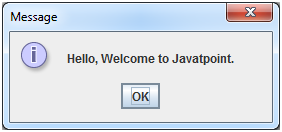
Java JOptionPane Example: showMessageDialog()
- import javax.swing.*;
- public class OptionPaneExample {
- JFrame f;
- OptionPaneExample(){
- f=new JFrame();
- JOptionPane.showMessageDialog(f,"Successfully Updated.","Alert",JOptionPane.WARNING_MESSAGE);
- }
- public static void main(String[] args) {
- new OptionPaneExample();
- }
- }
Output:
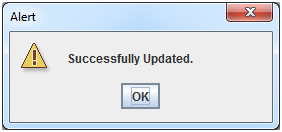
Java JOptionPane Example: showInputDialog()
- import javax.swing.*;
- public class OptionPaneExample {
- JFrame f;
- OptionPaneExample(){
- f=new JFrame();
- String name=JOptionPane.showInputDialog(f,"Enter Name");
- }
- public static void main(String[] args) {
- new OptionPaneExample();
- }
- }
Output:
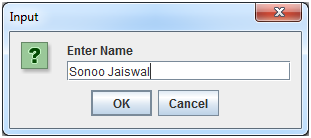
Java JOptionPane Example: showConfirmDialog()
- import javax.swing.*;
- import java.awt.event.*;
- public class OptionPaneExample extends WindowAdapter{
- JFrame f;
- OptionPaneExample(){
- f=new JFrame();
- f.addWindowListener(this);
- f.setSize(300, 300);
- f.setLayout(null);
- f.setDefaultCloseOperation(JFrame.DO_NOTHING_ON_CLOSE);
- f.setVisible(true);
- }
- public void windowClosing(WindowEvent e) {
- int a=JOptionPane.showConfirmDialog(f,"Are you sure?");
- if(a==JOptionPane.YES_OPTION){
- f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
- }
- }
- public static void main(String[] args) {
- new OptionPaneExample();
- }
- }
Output:
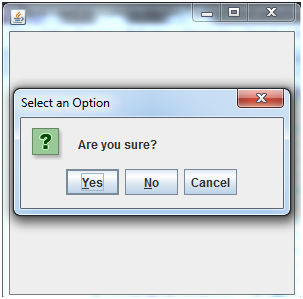
Java JScrollBar
The object of JScrollbar class is used to add horizontal and vertical scrollbar. It is an implementation of a scrollbar. It inherits JComponent class.
JScrollBar class declaration
Let's see the declaration for javax.swing.JScrollBar class.
- public class JScrollBar extends JComponent implements Adjustable, Accessible
Commonly used Constructors:
Constructor | Description |
---|
JScrollBar() | Creates a vertical scrollbar with the initial values. |
JScrollBar(int orientation) | Creates a scrollbar with the specified orientation and the initial values. |
JScrollBar(int orientation, int value, int extent, int min, int max) | Creates a scrollbar with the specified orientation, value, extent, minimum, and maximum. |
Java JScrollBar Example
- import javax.swing.*;
- class ScrollBarExample
- {
- ScrollBarExample(){
- JFrame f= new JFrame("Scrollbar Example");
- JScrollBar s=new JScrollBar();
- s.setBounds(100,100, 50,100);
- f.add(s);
- f.setSize(400,400);
- f.setLayout(null);
- f.setVisible(true);
- }
- public static void main(String args[])
- {
- new ScrollBarExample();
- }}
Output:
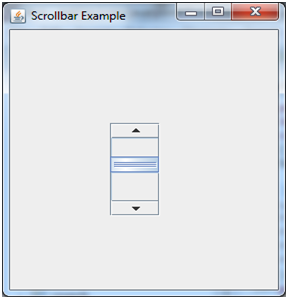
Java JScrollBar Example with AdjustmentListener
- import javax.swing.*;
- import java.awt.event.*;
- class ScrollBarExample
- {
- ScrollBarExample(){
- JFrame f= new JFrame("Scrollbar Example");
- final JLabel label = new JLabel();
- label.setHorizontalAlignment(JLabel.CENTER);
- label.setSize(400,100);
- final JScrollBar s=new JScrollBar();
- s.setBounds(100,100, 50,100);
- f.add(s); f.add(label);
- f.setSize(400,400);
- f.setLayout(null);
- f.setVisible(true);
- s.addAdjustmentListener(new AdjustmentListener() {
- public void adjustmentValueChanged(AdjustmentEvent e) {
- label.setText("Vertical Scrollbar value is:"+ s.getValue());
- }
- });
- }
- public static void main(String args[])
- {
- new ScrollBarExample();
- }}
Output:
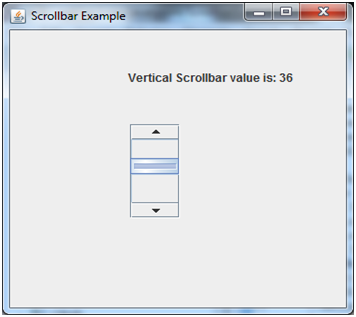
Anurag Rana
Educator CSE/IT
Comments
Post a Comment