Java JMenuBar, JMenu and JMenuItem
The JMenuBar class is used to display menubar on the window or frame. It may have several menus.
The object of JMenu class is a pull down menu component which is displayed from the menu bar. It inherits the JMenuItem class.
The object of JMenuItem class adds a simple labeled menu item. The items used in a menu must belong to the JMenuItem or any of its subclass.
JMenuBar class declaration
- public class JMenuBar extends JComponent implements MenuElement, Accessible
JMenu class declaration
- public class JMenu extends JMenuItem implements MenuElement, Accessible
JMenuItem class declaration
- public class JMenuItem extends AbstractButton implements Accessible, MenuElement
Java JMenuItem and JMenu Example
- import javax.swing.*;
- class MenuExample
- {
- JMenu menu, submenu;
- JMenuItem i1, i2, i3, i4, i5;
- MenuExample(){
- JFrame f= new JFrame("Menu and MenuItem Example");
- JMenuBar mb=new JMenuBar();
- menu=new JMenu("Menu");
- submenu=new JMenu("Sub Menu");
- i1=new JMenuItem("Item 1");
- i2=new JMenuItem("Item 2");
- i3=new JMenuItem("Item 3");
- i4=new JMenuItem("Item 4");
- i5=new JMenuItem("Item 5");
- menu.add(i1); menu.add(i2); menu.add(i3);
- submenu.add(i4); submenu.add(i5);
- menu.add(submenu);
- mb.add(menu);
- f.setJMenuBar(mb);
- f.setSize(400,400);
- f.setLayout(null);
- f.setVisible(true);
- }
- public static void main(String args[])
- {
- new MenuExample();
- }}
Output:

Example of creating Edit menu for Notepad:
- import javax.swing.*;
- import java.awt.event.*;
- public class MenuExample implements ActionListener{
- JFrame f;
- JMenuBar mb;
- JMenu file,edit,help;
- JMenuItem cut,copy,paste,selectAll;
- JTextArea ta;
- MenuExample(){
- f=new JFrame();
- cut=new JMenuItem("cut");
- copy=new JMenuItem("copy");
- paste=new JMenuItem("paste");
- selectAll=new JMenuItem("selectAll");
- cut.addActionListener(this);
- copy.addActionListener(this);
- paste.addActionListener(this);
- selectAll.addActionListener(this);
- mb=new JMenuBar();
- file=new JMenu("File");
- edit=new JMenu("Edit");
- help=new JMenu("Help");
- edit.add(cut);edit.add(copy);edit.add(paste);edit.add(selectAll);
- mb.add(file);mb.add(edit);mb.add(help);
- ta=new JTextArea();
- ta.setBounds(5,5,360,320);
- f.add(mb);f.add(ta);
- f.setJMenuBar(mb);
- f.setLayout(null);
- f.setSize(400,400);
- f.setVisible(true);
- }
- public void actionPerformed(ActionEvent e) {
- if(e.getSource()==cut)
- ta.cut();
- if(e.getSource()==paste)
- ta.paste();
- if(e.getSource()==copy)
- ta.copy();
- if(e.getSource()==selectAll)
- ta.selectAll();
- }
- public static void main(String[] args) {
- new MenuExample();
- }
- }
Output:
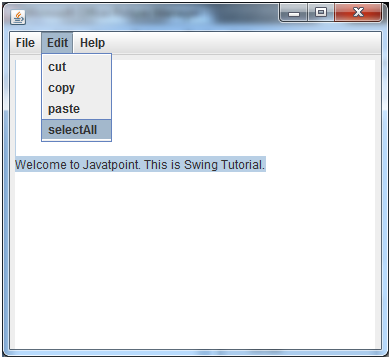
Java JPopupMenu
PopupMenu can be dynamically popped up at specific position within a component. It inherits the JComponent class.
JPopupMenu class declaration
Let's see the declaration for javax.swing.JPopupMenu class.
- public class JPopupMenu extends JComponent implements Accessible, MenuElement
Commonly used Constructors:
Constructor | Description |
---|
JPopupMenu() | Constructs a JPopupMenu without an "invoker". |
JPopupMenu(String label) | Constructs a JPopupMenu with the specified title. |
Java JPopupMenu Example
- import javax.swing.*;
- import java.awt.event.*;
- class PopupMenuExample
- {
- PopupMenuExample(){
- final JFrame f= new JFrame("PopupMenu Example");
- final JPopupMenu popupmenu = new JPopupMenu("Edit");
- JMenuItem cut = new JMenuItem("Cut");
- JMenuItem copy = new JMenuItem("Copy");
- JMenuItem paste = new JMenuItem("Paste");
- popupmenu.add(cut); popupmenu.add(copy); popupmenu.add(paste);
- f.addMouseListener(new MouseAdapter() {
- public void mouseClicked(MouseEvent e) {
- popupmenu.show(f , e.getX(), e.getY());
- }
- });
- f.add(popupmenu);
- f.setSize(300,300);
- f.setLayout(null);
- f.setVisible(true);
- }
- public static void main(String args[])
- {
- new PopupMenuExample();
- }}
Output:
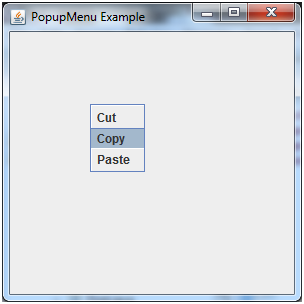
Java JPopupMenu Example with MouseListener and ActionListener
- import javax.swing.*;
- import java.awt.event.*;
- class PopupMenuExample
- {
- PopupMenuExample(){
- final JFrame f= new JFrame("PopupMenu Example");
- final JLabel label = new JLabel();
- label.setHorizontalAlignment(JLabel.CENTER);
- label.setSize(400,100);
- final JPopupMenu popupmenu = new JPopupMenu("Edit");
- JMenuItem cut = new JMenuItem("Cut");
- JMenuItem copy = new JMenuItem("Copy");
- JMenuItem paste = new JMenuItem("Paste");
- popupmenu.add(cut); popupmenu.add(copy); popupmenu.add(paste);
- f.addMouseListener(new MouseAdapter() {
- public void mouseClicked(MouseEvent e) {
- popupmenu.show(f , e.getX(), e.getY());
- }
- });
- cut.addActionListener(new ActionListener(){
- public void actionPerformed(ActionEvent e) {
- label.setText("cut MenuItem clicked.");
- }
- });
- copy.addActionListener(new ActionListener(){
- public void actionPerformed(ActionEvent e) {
- label.setText("copy MenuItem clicked.");
- }
- });
- paste.addActionListener(new ActionListener(){
- public void actionPerformed(ActionEvent e) {
- label.setText("paste MenuItem clicked.");
- }
- });
- f.add(label); f.add(popupmenu);
- f.setSize(400,400);
- f.setLayout(null);
- f.setVisible(true);
- }
- public static void main(String args[])
- {
- new PopupMenuExample();
- }
- }
Output:
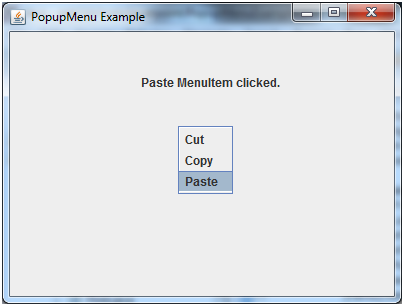
Java JCheckBoxMenuItem
JCheckBoxMenuItem class represents checkbox which can be included on a menu . A CheckBoxMenuItem can have text or a graphic icon or both, associated with it. MenuItem can be selected or deselected. MenuItems can be configured and controlled by actions.
Nested class
Modifier and Type | Class | Description |
---|
protected class | JCheckBoxMenuItem.AccessibleJCheckBoxMenuItem | This class implements accessibility support for the JcheckBoxMenuItem class. |
Constructor
Constructor | Description |
---|
JCheckBoxMenuItem() | It creates an initially unselected check box menu item with no set text or icon. |
JCheckBoxMenuItem(Action a) | It creates a menu item whose properties are taken from the Action supplied. |
JCheckBoxMenuItem(Icon icon) | It creates an initially unselected check box menu item with an icon. |
JCheckBoxMenuItem(String text) | It creates an initially unselected check box menu item with text. |
JCheckBoxMenuItem(String text, boolean b) | It creates a check box menu item with the specified text and selection state. |
JCheckBoxMenuItem(String text, Icon icon) | It creates an initially unselected check box menu item with the specified text and icon. |
JCheckBoxMenuItem(String text, Icon icon, boolean b) | It creates a check box menu item with the specified text, icon, and selection state. |
Methods
Modifier | Method | Description |
---|
AccessibleContext | getAccessibleContext() | It gets the AccessibleContext associated with this JCheckBoxMenuItem. |
Object[] | getSelectedObjects() | It returns an array (length 1) containing the check box menu item label or null if the check box is not selected. |
boolean | getState() | It returns the selected-state of the item. |
String | getUIClassID() | It returns the name of the L&F class that renders this component. |
protected String | paramString() | It returns a string representation of this JCheckBoxMenuItem. |
void | setState(boolean b) | It sets the selected-state of the item. |
Java JCheckBoxMenuItem Example
- import java.awt.event.ActionEvent;
- import java.awt.event.ActionListener;
- import java.awt.event.KeyEvent;
- import javax.swing.AbstractButton;
- import javax.swing.Icon;
- import javax.swing.JCheckBoxMenuItem;
- import javax.swing.JFrame;
- import javax.swing.JMenu;
- import javax.swing.JMenuBar;
- import javax.swing.JMenuItem;
-
- public class JavaCheckBoxMenuItem {
- public static void main(final String args[]) {
- JFrame frame = new JFrame("Jmenu Example");
- frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
- JMenuBar menuBar = new JMenuBar();
-
- JMenu fileMenu = new JMenu("File");
- fileMenu.setMnemonic(KeyEvent.VK_F);
- menuBar.add(fileMenu);
-
- JMenuItem menuItem1 = new JMenuItem("Open", KeyEvent.VK_N);
- fileMenu.add(menuItem1);
-
- JCheckBoxMenuItem caseMenuItem = new JCheckBoxMenuItem("Option_1");
- caseMenuItem.setMnemonic(KeyEvent.VK_C);
- fileMenu.add(caseMenuItem);
-
- ActionListener aListener = new ActionListener() {
- public void actionPerformed(ActionEvent event) {
- AbstractButton aButton = (AbstractButton) event.getSource();
- boolean selected = aButton.getModel().isSelected();
- String newLabel;
- Icon newIcon;
- if (selected) {
- newLabel = "Value-1";
- } else {
- newLabel = "Value-2";
- }
- aButton.setText(newLabel);
- }
- };
-
- caseMenuItem.addActionListener(aListener);
- frame.setJMenuBar(menuBar);
- frame.setSize(350, 250);
- frame.setVisible(true);
- }
- }
Output:
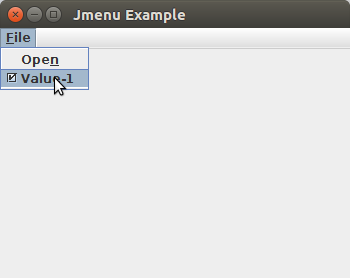
Anurag Rana
Educator CSE/IT
Comments
Post a Comment