Java JDialog
The JDialog control represents a top level window with a border and a title used to take some form of input from the user. It inherits the Dialog class.
Unlike JFrame, it doesn't have maximize and minimize buttons.
JDialog class declaration
Let's see the declaration for javax.swing.JDialog class.
Commonly used Constructors:
Constructor | Description |
---|---|
JDialog() | It is used to create a modeless dialog without a title and without a specified Frame owner. |
JDialog(Frame owner) | It is used to create a modeless dialog with specified Frame as its owner and an empty title. |
JDialog(Frame owner, String title, boolean modal) | It is used to create a dialog with the specified title, owner Frame and modality. |
Java JDialog Example
Output:
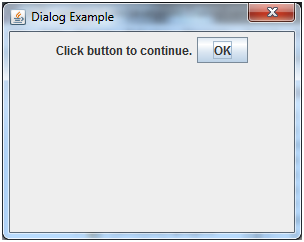
Java JPanel
The JPanel is a simplest container class. It provides space in which an application can attach any other component. It inherits the JComponents class.
It doesn't have title bar.
JPanel class declaration
Commonly used Constructors:
Constructor | Description |
---|---|
JPanel() | It is used to create a new JPanel with a double buffer and a flow layout. |
JPanel(boolean isDoubleBuffered) | It is used to create a new JPanel with FlowLayout and the specified buffering strategy. |
JPanel(LayoutManager layout) | It is used to create a new JPanel with the specified layout manager. |
Java JPanel Example
Output:
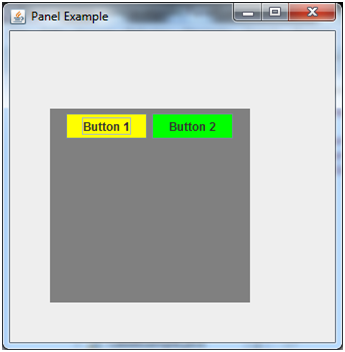
The object of JFileChooser class represents a dialog window from which the user can select file. It inherits JComponent class.
JFileChooser class declaration
Let's see the declaration for javax.swing.JFileChooser class.
Commonly used Constructors:
Constructor | Description |
---|---|
JFileChooser() | Constructs a JFileChooser pointing to the user's default directory. |
JFileChooser(File currentDirectory) | Constructs a JFileChooser using the given File as the path. |
JFileChooser(String currentDirectoryPath) | Constructs a JFileChooser using the given path. |
Java JFileChooser Example
Output:
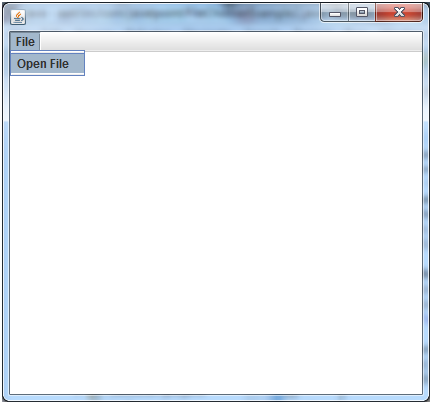
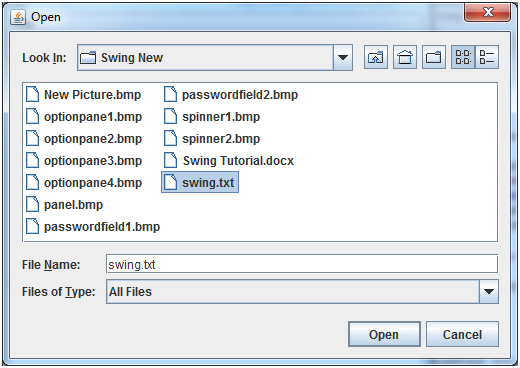
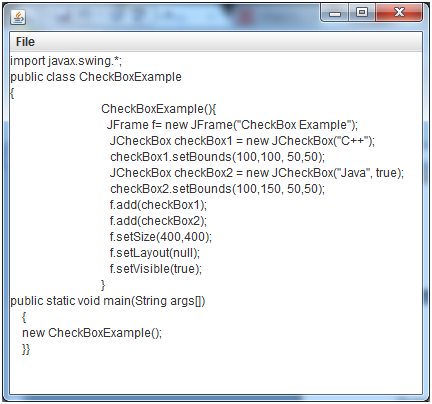
Java JToggleButton
JToggleButton is used to create toggle button, it is two-states button to switch on or off.
Nested Classes
Modifier and Type | Class | Description |
---|---|---|
protected class | JToggleButton.AccessibleJToggleButton | This class implements accessibility support for the JToggleButton class. |
static class | JToggleButton.ToggleButtonModel | The ToggleButton model |
Constructors
Constructor | Description |
---|---|
JToggleButton() | It creates an initially unselected toggle button without setting the text or image. |
JToggleButton(Action a) | It creates a toggle button where properties are taken from the Action supplied. |
JToggleButton(Icon icon) | It creates an initially unselected toggle button with the specified image but no text. |
JToggleButton(Icon icon, boolean selected) | It creates a toggle button with the specified image and selection state, but no text. |
JToggleButton(String text) | It creates an unselected toggle button with the specified text. |
JToggleButton(String text, boolean selected) | It creates a toggle button with the specified text and selection state. |
JToggleButton(String text, Icon icon) | It creates a toggle button that has the specified text and image, and that is initially unselected. |
JToggleButton(String text, Icon icon, boolean selected) | It creates a toggle button with the specified text, image, and selection state. |
Methods
Modifier and Type | Method | Description |
---|---|---|
AccessibleContext | getAccessibleContext() | It gets the AccessibleContext associated with this JToggleButton. |
String | getUIClassID() | It returns a string that specifies the name of the l&f class that renders this component. |
protected String | paramString() | It returns a string representation of this JToggleButton. |
void | updateUI() | It resets the UI property to a value from the current look and feel. |
JToggleButton Example
Output
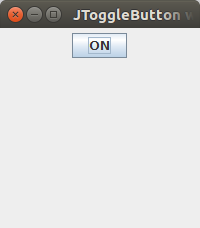
Comments
Post a Comment