Java JColorChooser
The JColorChooser class is used to create a color chooser dialog box so that user can select any color. It inherits JComponent class.
JColorChooser class declaration
Let's see the declaration for javax.swing.JColorChooser class.
- public class JColorChooser extends JComponent implements Accessible
Commonly used Constructors:
Constructor | Description |
---|
JColorChooser() | It is used to create a color chooser panel with white color initially. |
JColorChooser(color initialcolor) | It is used to create a color chooser panel with the specified color initially. |
Commonly used Methods:
Method | Description |
---|
void addChooserPanel(AbstractColorChooserPanel panel) | It is used to add a color chooser panel to the color chooser. |
static Color showDialog(Component c, String title, Color initialColor) | It is used to show the color chooser dialog box. |
Java JColorChooser Example
- import java.awt.event.*;
- import java.awt.*;
- import javax.swing.*;
- public class ColorChooserExample extends JFrame implements ActionListener {
- JButton b;
- Container c;
- ColorChooserExample(){
- c=getContentPane();
- c.setLayout(new FlowLayout());
- b=new JButton("color");
- b.addActionListener(this);
- c.add(b);
- }
- public void actionPerformed(ActionEvent e) {
- Color initialcolor=Color.RED;
- Color color=JColorChooser.showDialog(this,"Select a color",initialcolor);
- c.setBackground(color);
- }
-
- public static void main(String[] args) {
- ColorChooserExample ch=new ColorChooserExample();
- ch.setSize(400,400);
- ch.setVisible(true);
- ch.setDefaultCloseOperation(EXIT_ON_CLOSE);
- }
- }
Output:
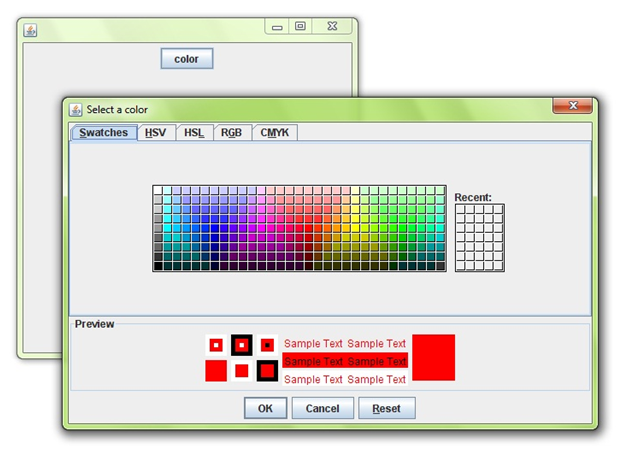
Java JColorChooser Example with ActionListener
- import javax.swing.*;
- import java.awt.*;
- import java.awt.event.*;
- public class ColorChooserExample extends JFrame implements ActionListener{
- JFrame f;
- JButton b;
- JTextArea ta;
- ColorChooserExample(){
- f=new JFrame("Color Chooser Example.");
- b=new JButton("Pad Color");
- b.setBounds(200,250,100,30);
- ta=new JTextArea();
- ta.setBounds(10,10,300,200);
- b.addActionListener(this);
- f.add(b);f.add(ta);
- f.setLayout(null);
- f.setSize(400,400);
- f.setVisible(true);
- }
- public void actionPerformed(ActionEvent e){
- Color c=JColorChooser.showDialog(this,"Choose",Color.CYAN);
- ta.setBackground(c);
- }
- public static void main(String[] args) {
- new ColorChooserExample();
- }
- }
Output:
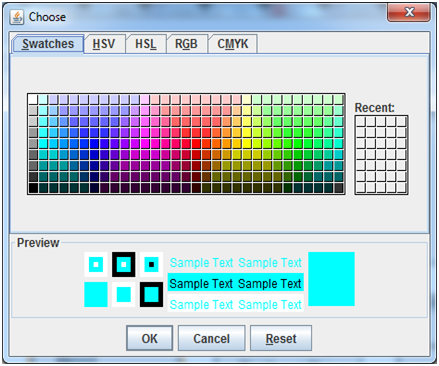
Java JTabbedPane
The JTabbedPane class is used to switch between a group of components by clicking on a tab with a given title or icon. It inherits JComponent class.
JTabbedPane class declaration
Let's see the declaration for javax.swing.JTabbedPane class.
- public class JTabbedPane extends JComponent implements Serializable, Accessible, SwingConstants
Commonly used Constructors:
Constructor | Description |
---|
JTabbedPane() | Creates an empty TabbedPane with a default tab placement of JTabbedPane.Top. |
JTabbedPane(int tabPlacement) | Creates an empty TabbedPane with a specified tab placement. |
JTabbedPane(int tabPlacement, int tabLayoutPolicy) | Creates an empty TabbedPane with a specified tab placement and tab layout policy. |
Java JTabbedPane Example
- import javax.swing.*;
- public class TabbedPaneExample {
- JFrame f;
- TabbedPaneExample(){
- f=new JFrame();
- JTextArea ta=new JTextArea(200,200);
- JPanel p1=new JPanel();
- p1.add(ta);
- JPanel p2=new JPanel();
- JPanel p3=new JPanel();
- JTabbedPane tp=new JTabbedPane();
- tp.setBounds(50,50,200,200);
- tp.add("main",p1);
- tp.add("visit",p2);
- tp.add("help",p3);
- f.add(tp);
- f.setSize(400,400);
- f.setLayout(null);
- f.setVisible(true);
- }
- public static void main(String[] args) {
- new TabbedPaneExample();
- }}
Output:
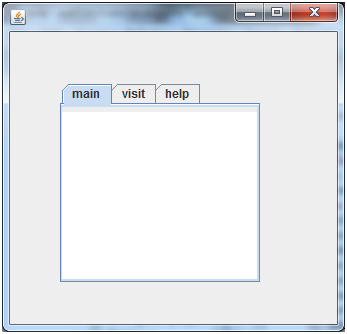
Java JSlider
The Java JSlider class is used to create the slider. By using JSlider, a user can select a value from a specific range.
Commonly used Constructors of JSlider class
Constructor | Description |
---|
JSlider() | creates a slider with the initial value of 50 and range of 0 to 100. |
JSlider(int orientation) | creates a slider with the specified orientation set by either JSlider.HORIZONTAL or JSlider.VERTICAL with the range 0 to 100 and initial value 50. |
JSlider(int min, int max) | creates a horizontal slider using the given min and max. |
JSlider(int min, int max, int value) | creates a horizontal slider using the given min, max and value. |
JSlider(int orientation, int min, int max, int value) | creates a slider using the given orientation, min, max and value. |
Commonly used Methods of JSlider class
Method | Description |
---|
public void setMinorTickSpacing(int n) | is used to set the minor tick spacing to the slider. |
public void setMajorTickSpacing(int n) | is used to set the major tick spacing to the slider. |
public void setPaintTicks(boolean b) | is used to determine whether tick marks are painted. |
public void setPaintLabels(boolean b) | is used to determine whether labels are painted. |
public void setPaintTracks(boolean b) | is used to determine whether track is painted. |
Java JSlider Example
- import javax.swing.*;
- public class SliderExample1 extends JFrame{
- public SliderExample1() {
- JSlider slider = new JSlider(JSlider.HORIZONTAL, 0, 50, 25);
- JPanel panel=new JPanel();
- panel.add(slider);
- add(panel);
- }
-
- public static void main(String s[]) {
- SliderExample1 frame=new SliderExample1();
- frame.pack();
- frame.setVisible(true);
- }
- }
Output:
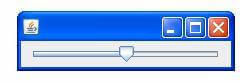
Java JSlider Example: painting ticks
- import javax.swing.*;
- public class SliderExample extends JFrame{
- public SliderExample() {
- JSlider slider = new JSlider(JSlider.HORIZONTAL, 0, 50, 25);
- slider.setMinorTickSpacing(2);
- slider.setMajorTickSpacing(10);
- slider.setPaintTicks(true);
- slider.setPaintLabels(true);
-
- JPanel panel=new JPanel();
- panel.add(slider);
- add(panel);
- }
- public static void main(String s[]) {
- SliderExample frame=new SliderExample();
- frame.pack();
- frame.setVisible(true);
- }
- }
Output:
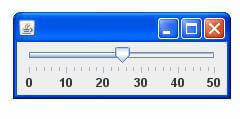
Java JSpinner
The object of JSpinner class is a single line input field that allows the user to select a number or an object value from an ordered sequence.
JSpinner class declaration
Let's see the declaration for javax.swing.JSpinner class.
- public class JSpinner extends JComponent implements Accessible
Commonly used Contructors:
Constructor | Description |
---|
JSpinner() | It is used to construct a spinner with an Integer SpinnerNumberModel with initial value 0 and no minimum or maximum limits. |
JSpinner(SpinnerModel model) | It is used to construct a spinner for a given model. |
Commonly used Methods:
Method | Description |
---|
void addChangeListener(ChangeListener listener) | It is used to add a listener to the list that is notified each time a change to the model occurs. |
Object getValue() | It is used to return the current value of the model. |
Java JSpinner Example
- import javax.swing.*;
- public class SpinnerExample {
- public static void main(String[] args) {
- JFrame f=new JFrame("Spinner Example");
- SpinnerModel value =
- new SpinnerNumberModel(5,
- 0,
- 10,
- 1);
- JSpinner spinner = new JSpinner(value);
- spinner.setBounds(100,100,50,30);
- f.add(spinner);
- f.setSize(300,300);
- f.setLayout(null);
- f.setVisible(true);
- }
- }
Output:
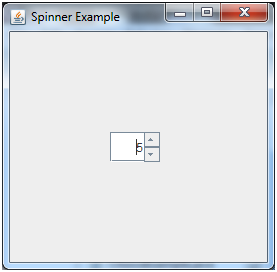
Java JSpinner Example with ChangeListener
imp- ort javax.swing.*;
- import javax.swing.event.*;
- public class SpinnerExample {
- public static void main(String[] args) {
- JFrame f=new JFrame("Spinner Example");
- final JLabel label = new JLabel();
- label.setHorizontalAlignment(JLabel.CENTER);
- label.setSize(250,100);
- SpinnerModel value =
- new SpinnerNumberModel(5,
- 0,
- 10,
- 1);
- JSpinner spinner = new JSpinner(value);
- spinner.setBounds(100,100,50,30);
- f.add(spinner); f.add(label);
- f.setSize(300,300);
- f.setLayout(null);
- f.setVisible(true);
- spinner.addChangeListener(new ChangeListener() {
- public void stateChanged(ChangeEvent e) {
- label.setText("Value : " + ((JSpinner)e.getSource()).getValue());
- }
- });
- }
- }
Output:
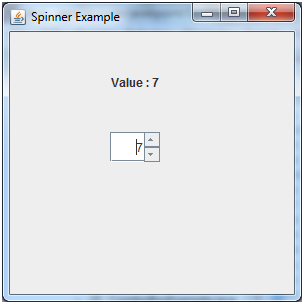
Anurag Rana
Educator CSE/IT
Comments
Post a Comment