ava AWT Scrollbar
The object of Scrollbar class is used to add horizontal and vertical scrollbar. Scrollbar is a GUI component allows us to see invisible number of rows and columns.
AWT Scrollbar class declaration
- public class Scrollbar extends Component implements Adjustable, Accessible
Java AWT Scrollbar Example
- import java.awt.*;
- class ScrollbarExample{
- ScrollbarExample(){
- Frame f= new Frame("Scrollbar Example");
- Scrollbar s=new Scrollbar();
- s.setBounds(100,100, 50,100);
- f.add(s);
- f.setSize(400,400);
- f.setLayout(null);
- f.setVisible(true);
- }
- public static void main(String args[]){
- new ScrollbarExample();
- }
- }
Output:
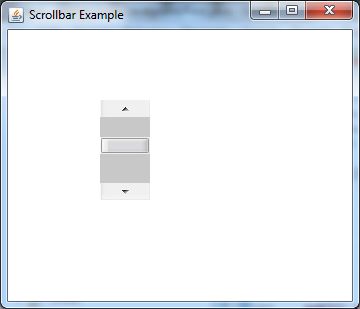
Java AWT Scrollbar Example with AdjustmentListener
- import java.awt.*;
- import java.awt.event.*;
- class ScrollbarExample{
- ScrollbarExample(){
- Frame f= new Frame("Scrollbar Example");
- final Label label = new Label();
- label.setAlignment(Label.CENTER);
- label.setSize(400,100);
- final Scrollbar s=new Scrollbar();
- s.setBounds(100,100, 50,100);
- f.add(s);f.add(label);
- f.setSize(400,400);
- f.setLayout(null);
- f.setVisible(true);
- s.addAdjustmentListener(new AdjustmentListener() {
- public void adjustmentValueChanged(AdjustmentEvent e) {
- label.setText("Vertical Scrollbar value is:"+ s.getValue());
- }
- });
- }
- public static void main(String args[]){
- new ScrollbarExample();
- }
- }
Output:
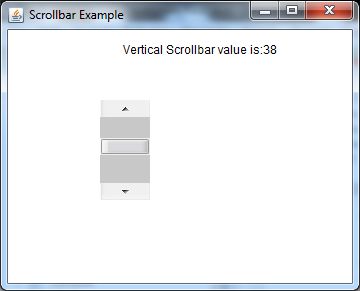
Java AWT MenuItem and Menu
The object of MenuItem class adds a simple labeled menu item on menu. The items used in a menu must belong to the MenuItem or any of its subclass.
The object of Menu class is a pull down menu component which is displayed on the menu bar. It inherits the MenuItem class.
AWT MenuItem class declaration
- public class MenuItem extends MenuComponent implements Accessible
AWT Menu class declaration
- public class Menu extends MenuItem implements MenuContainer, Accessible
Java AWT MenuItem and Menu Example
- import java.awt.*;
- class MenuExample
- {
- MenuExample(){
- Frame f= new Frame("Menu and MenuItem Example");
- MenuBar mb=new MenuBar();
- Menu menu=new Menu("Menu");
- Menu submenu=new Menu("Sub Menu");
- MenuItem i1=new MenuItem("Item 1");
- MenuItem i2=new MenuItem("Item 2");
- MenuItem i3=new MenuItem("Item 3");
- MenuItem i4=new MenuItem("Item 4");
- MenuItem i5=new MenuItem("Item 5");
- menu.add(i1);
- menu.add(i2);
- menu.add(i3);
- submenu.add(i4);
- submenu.add(i5);
- menu.add(submenu);
- mb.add(menu);
- f.setMenuBar(mb);
- f.setSize(400,400);
- f.setLayout(null);
- f.setVisible(true);
- }
- public static void main(String args[])
- {
- new MenuExample();
- }
- }
Output:

ava AWT PopupMenu
PopupMenu can be dynamically popped up at specific position within a component. It inherits the Menu class.
AWT PopupMenu class declaration
- public class PopupMenu extends Menu implements MenuContainer, Accessible
Java AWT PopupMenu Example
- import java.awt.*;
- import java.awt.event.*;
- class PopupMenuExample
- {
- PopupMenuExample(){
- final Frame f= new Frame("PopupMenu Example");
- final PopupMenu popupmenu = new PopupMenu("Edit");
- MenuItem cut = new MenuItem("Cut");
- cut.setActionCommand("Cut");
- MenuItem copy = new MenuItem("Copy");
- copy.setActionCommand("Copy");
- MenuItem paste = new MenuItem("Paste");
- paste.setActionCommand("Paste");
- popupmenu.add(cut);
- popupmenu.add(copy);
- popupmenu.add(paste);
- f.addMouseListener(new MouseAdapter() {
- public void mouseClicked(MouseEvent e) {
- popupmenu.show(f , e.getX(), e.getY());
- }
- });
- f.add(popupmenu);
- f.setSize(400,400);
- f.setLayout(null);
- f.setVisible(true);
- }
- public static void main(String args[])
- {
- new PopupMenuExample();
- }
- }
Output:
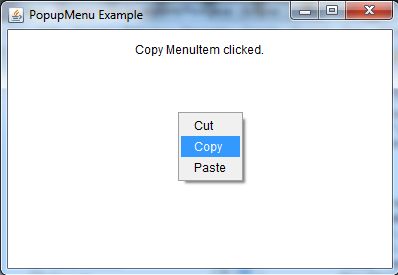
Anurag Rana
Educator CSE/IT
Comments
Post a Comment