C++ Functions
The function in C++ language is also known as procedure or subroutine in other programming languages.
To perform any task, we can create function. A function can be called many times. It provides modularity and code reusability.
Advantage of functions in C
There are many advantages of functions.
1) Code Reusability
By creating functions in C++, you can call it many times. So we don't need to write the same code again and again.
2) Code optimization
It makes the code optimized, we don't need to write much code.
Suppose, you have to check 3 numbers (531, 883 and 781) whether it is prime number or not. Without using function, you need to write the prime number logic 3 times. So, there is repetition of code.
But if you use functions, you need to write the logic only once and you can reuse it several times.
Types of Functions
There are two types of functions in C programming:
1. Library Functions: are the functions which are declared in the C++ header files such as ceil(x), cos(x), exp(x), etc.
2. User-defined functions: are the functions which are created by the C++ programmer, so that he/she can use it many times. It reduces complexity of a big program and optimizes the code.
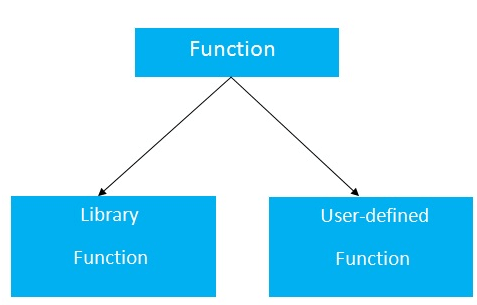
Declaration of a function
The syntax of creating function in C++ language is given below:
C++ Function Example
Let's see the simple example of C++ function.
Output:
i= 1 and j= 1 i= 2 and j= 1 i= 3 and j= 1
Call by value and call by reference in C++
There are two ways to pass value or data to function in C language: call by value and call by reference. Original value is not modified in call by value but it is modified in call by reference.

Let's understand call by value and call by reference in C++ language one by one.
Call by value in C++
In call by value, original value is not modified.
In call by value, value being passed to the function is locally stored by the function parameter in stack memory location. If you change the value of function parameter, it is changed for the current function only. It will not change the value of variable inside the caller method such as main().
Let's try to understand the concept of call by value in C++ language by the example given below:
Output:
Value of the data is: 3
Call by reference in C++
In call by reference, original value is modified because we pass reference (address).
Here, address of the value is passed in the function, so actual and formal arguments share the same address space. Hence, value changed inside the function, is reflected inside as well as outside the function.
Note: To understand the call by reference, you must have the basic knowledge of pointers.
Let's try to understand the concept of call by reference in C++ language by the example given below:
Output:
Value of x is: 100 Value of y is: 500
Difference between call by value and call by reference in C++
No. | Call by value | Call by reference |
---|---|---|
1 | A copy of value is passed to the function | An address of value is passed to the function |
2 | Changes made inside the function is not reflected on other functions | Changes made inside the function is reflected outside the function also |
3 | Actual and formal arguments will be created in different memory location | Actual and formal arguments will be created in same memory location |
Comments
Post a Comment