Java JEditorPane
JEditorPane class is used to create a simple text editor window. This class has setContentType() and setText() methods.
setContentType("text/plain"): This method is used to set the content type to be plain text.
setText(text): This method is used to set the initial text content.
Nested Classes
Modifier and Type | Class | Description |
---|
protected class | JEditorPane.AccessibleJEditorPane | This class implements accessibility support for the JEditorPane class. |
protected class | JEditorPane.AccessibleJEditorPaneHTML | This class provides support for AccessibleHypertext, and is used in instances where the EditorKit installed in this JEditorPane is an instance of HTMLEditorKit. |
protected class | JEditorPane.JEditorPaneAccessibleHypertextSupport | What's returned by AccessibleJEditorPaneHTML.getAccessibleText |
Fields
Modifier and Type | Field | Description |
---|
static String | HONOR_DISPLAY_PROPERTIES | Key for a client property used to indicate whether the default font and foreground color from the component are used if a font or foreground color is not specified in the styled text. |
static String | W3C_LENGTH_UNITS | Key for a client property used to indicate whether w3c compliant length units are used for html rendering. |
Constructors
Constructor | Description |
---|
JEditorPane() | It creates a new JEditorPane. |
JEditorPane(String url) | It creates a JEditorPane based on a string containing a URL specification. |
JEditorPane(String type, String text) | It creates a JEditorPane that has been initialized to the given text. |
JEditorPane(URL initialPage) | It creates a JEditorPane based on a specified URL for input. |
Useful Methods
Modifier and Type | Method | Description |
---|
void | addHyperlinkListener(HyperlinkListener listener) | Adds a hyperlink listener for notification of any changes, for example when a link is selected and entered. |
protected EditorKit | createDefaultEditorKit() | It creates the default editor kit (PlainEditorKit) for when the component is first created. |
void | setText(String t) | It sets the text of this TextComponent to the specified content, which is expected to be in the format of the content type of this editor. |
void | setContentType(String type) | It sets the type of content that this editor handles. |
void | setPage(URL page) | It sets the current URL being displayed. |
void | read(InputStream in, Object desc) | This method initializes from a stream. |
void | scrollToReference(String reference) | It scrolls the view to the given reference location (that is, the value returned by the UL.getRef method for the URL being displayed). |
void | setText(String t) | It sets the text of this TextComponent to the specified content, which is expected to be in the format of the content type of this editor. |
String | getText() | It returns the text contained in this TextComponent in terms of the content type of this editor. |
void | read(InputStream in, Object desc) | This method initializes from a stream. |
JEditorPane Example
- import javax.swing.JEditorPane;
- import javax.swing.JFrame;
-
- public class JEditorPaneExample {
- JFrame myFrame = null;
-
- public static void main(String[] a) {
- (new JEditorPaneExample()).test();
- }
-
- private void test() {
- myFrame = new JFrame("JEditorPane Test");
- myFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
- myFrame.setSize(400, 200);
- JEditorPane myPane = new JEditorPane();
- myPane.setContentType("text/plain");
- myPane.setText("Sleeping is necessary for a healthy body."
- + " But sleeping in unnecessary times may spoil our health, wealth and studies."
- + " Doctors advise that the sleeping at improper timings may lead for obesity during the students days.");
- myFrame.setContentPane(myPane);
- myFrame.setVisible(true);
- }
- }
Output:
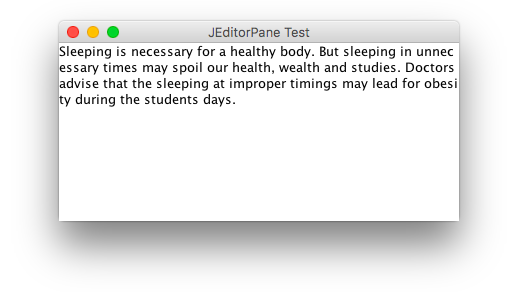
JEditorPane Example: using HTML
- import javax.swing.JEditorPane;
- import javax.swing.JFrame;
-
- public class JEditorPaneExample {
- JFrame myFrame = null;
-
- public static void main(String[] a) {
- (new JEditorPaneExample()).test();
- }
-
- private void test() {
- myFrame = new JFrame("JEditorPane Test");
- myFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
- myFrame.setSize(400, 200);
- JEditorPane myPane = new JEditorPane();
- myPane.setContentType("text/html");
- myPane.setText("<h1>Sleeping</h1><p>Sleeping is necessary for a healthy body."
- + " But sleeping in unnecessary times may spoil our health, wealth and studies."
- + " Doctors advise that the sleeping at improper timings may lead for obesity during the students days.</p>");
- myFrame.setContentPane(myPane);
- myFrame.setVisible(true);
- }
- }
Output:
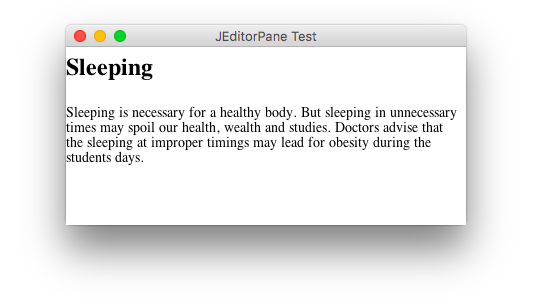
Java JScrollPane
A JscrollPane is used to make scrollable view of a component. When screen size is limited, we use a scroll pane to display a large component or a component whose size can change dynamically.
Constructors
Constructor | Purpose |
---|
JScrollPane() | It creates a scroll pane. The Component parameter, when present, sets the scroll pane's client. The two int parameters, when present, set the vertical and horizontal scroll bar policies (respectively). |
JScrollPane(Component) |
JScrollPane(int, int) |
JScrollPane(Component, int, int) |
Useful Methods
Modifier | Method | Description |
---|
void | setColumnHeaderView(Component) | It sets the column header for the scroll pane. |
void | setRowHeaderView(Component) | It sets the row header for the scroll pane. |
void | setCorner(String, Component) | It sets or gets the specified corner. The int parameter specifies which corner and must be one of the following constants defined in ScrollPaneConstants: UPPER_LEFT_CORNER, UPPER_RIGHT_CORNER, LOWER_LEFT_CORNER, LOWER_RIGHT_CORNER, LOWER_LEADING_CORNER, LOWER_TRAILING_CORNER, UPPER_LEADING_CORNER, UPPER_TRAILING_CORNER. |
Component | getCorner(String) |
void | setViewportView(Component) | Set the scroll pane's client. |
JScrollPane Example
- import java.awt.FlowLayout;
- import javax.swing.JFrame;
- import javax.swing.JScrollPane;
- import javax.swing.JtextArea;
-
- public class JScrollPaneExample {
- private static final long serialVersionUID = 1L;
-
- private static void createAndShowGUI() {
-
-
- final JFrame frame = new JFrame("Scroll Pane Example");
-
-
- frame.setSize(500, 500);
- frame.setVisible(true);
- frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
-
-
- frame.getContentPane().setLayout(new FlowLayout());
-
- JTextArea textArea = new JTextArea(20, 20);
- JScrollPane scrollableTextArea = new JScrollPane(textArea);
-
- scrollableTextArea.setHorizontalScrollBarPolicy(JScrollPane.HORIZONTAL_SCROLLBAR_ALWAYS);
- scrollableTextArea.setVerticalScrollBarPolicy(JScrollPane.VERTICAL_SCROLLBAR_ALWAYS);
-
- frame.getContentPane().add(scrollableTextArea);
- }
- public static void main(String[] args) {
-
-
- javax.swing.SwingUtilities.invokeLater(new Runnable() {
-
- public void run() {
- createAndShowGUI();
- }
- });
- }
- }
Output:

Java JSplitPane
JSplitPane is used to divide two components. The two components are divided based on the look and feel implementation, and they can be resized by the user. If the minimum size of the two components is greater than the size of the split pane, the divider will not allow you to resize it.
The two components in a split pane can be aligned left to right using JSplitPane.HORIZONTAL_SPLIT, or top to bottom using JSplitPane.VERTICAL_SPLIT. When the user is resizing the components the minimum size of the components is used to determine the maximum/minimum position the components can be set to.
Nested Class
Modifier and Type | Class | Description |
---|
protected class | JSplitPane.AccessibleJSplitPane | This class implements accessibility support for the JsplitPane class. |
Useful Fields
Modifier and Type | Field | Description |
---|
static String | BOTTOM | It use to add a Component below the other Component. |
static String | CONTINUOUS_LAYOUT_PROPERTY | Bound property name for continuousLayout. |
static String | DIVIDER | It uses to add a Component that will represent the divider. |
static int | HORIZONTAL_SPLIT | Horizontal split indicates the Components are split along the x axis. |
protected int | lastDividerLocation | Previous location of the split pane. |
protected Component | leftComponent | The left or top component. |
static int | VERTICAL_SPLIT | Vertical split indicates the Components are split along the y axis. |
protected Component | rightComponent | The right or bottom component. |
protected int | orientation | How the views are split. |
Constructors
Constructor | Description |
JSplitPane() | It creates a new JsplitPane configured to arrange the child components side-by-side horizontally, using two buttons for the components. |
JSplitPane(int newOrientation) | It creates a new JsplitPane configured with the specified orientation. |
JSplitPane(int newOrientation, boolean newContinuousLayout) | It creates a new JsplitPane with the specified orientation and redrawing style. |
JSplitPane(int newOrientation, boolean newContinuousLayout, Component newLeftComponent, Component newRightComponent) | It creates a new JsplitPane with the specified orientation and redrawing style, and with the specified components. |
JSplitPane(int newOrientation, Component newLeftComponent, Component newRightComponent) | It creates a new JsplitPane with the specified orientation and the specified components. |
Useful Methods
Modifier and Type | Method | Description |
---|
protected void | addImpl(Component comp, Object constraints, int index) | It cdds the specified component to this split pane. |
AccessibleContext | getAccessibleContext() | It gets the AccessibleContext associated with this JSplitPane. |
int | getDividerLocation() | It returns the last value passed to setDividerLocation. |
int | getDividerSize() | It returns the size of the divider. |
Component | getBottomComponent() | It returns the component below, or to the right of the divider. |
Component | getRightComponent() | It returns the component to the right (or below) the divider. |
SplitPaneUI | getUI() | It returns the SplitPaneUI that is providing the current look and feel. |
boolean | isContinuousLayout() | It gets the continuousLayout property. |
boolean | isOneTouchExpandable() | It gets the oneTouchExpandable property. |
void | setOrientation(int orientation) | It gets the orientation, or how the splitter is divided. |
JSplitPane Example
- import java.awt.FlowLayout;
- import java.awt.Panel;
- import javax.swing.JComboBox;
- import javax.swing.JFrame;
- import javax.swing.JSplitPane;
- public class JSplitPaneExample {
- private static void createAndShow() {
-
- final JFrame frame = new JFrame("JSplitPane Example");
-
- frame.setSize(300, 300);
- frame.setVisible(true);
- frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
-
- frame.getContentPane().setLayout(new FlowLayout());
- String[] option1 = { "A","B","C","D","E" };
- JComboBox box1 = new JComboBox(option1);
- String[] option2 = {"1","2","3","4","5"};
- JComboBox box2 = new JComboBox(option2);
- Panel panel1 = new Panel();
- panel1.add(box1);
- Panel panel2 = new Panel();
- panel2.add(box2);
- JSplitPane splitPane = new JSplitPane(JSplitPane.HORIZONTAL_SPLIT, panel1, panel2);
-
-
- frame.getContentPane().add(splitPane);
- }
- public static void main(String[] args) {
-
-
- javax.swing.SwingUtilities.invokeLater(new Runnable() {
- public void run() {
- createAndShow();
- }
- });
- }
- }
Java JTextPane
JTextPane is a subclass of JEditorPane class. JTextPane is used for styled document with embedded images and components. It is text component that can be marked up with attributes that are represented graphically. JTextPane uses a DefaultStyledDocument as its default model.
Constructors
Constructor | Description |
---|
JTextPane() | It creates a new JTextPane. |
JtextPane(StyledDocument doc) | It creates a new JTextPane, with a specified document model. |
Useful Methods
Modifier and Type | Method | Description |
---|
Style | addStyle(String nm, Style parent) | It adds a new style into the logical style hierarchy. |
AttributeSet | getCharacterAttributes() | It fetches the character attributes in effect at the current location of the caret, or null. |
StyledDocument | getStyledDocument() | It fetches the model associated with the editor. |
void | setDocument(Document doc) | It associates the editor with a text document. |
void | setCharacterAttributes(AttributeSet attr, boolean replace) | It applies the given attributes to character content. |
void | removeStyle(String nm) | It removes a named non-null style previously added to the document. |
void | setEditorKit(EditorKit kit) | It sets the currently installed kit for handling content. |
void | setStyledDocument(StyledDocument doc) | It associates the editor with a text document. |
JTextPane Example
- import java.awt.BorderLayout;
- import java.awt.Color;
- import java.awt.Container;
- import javax.swing.JFrame;
- import javax.swing.JScrollPane;
- import javax.swing.JTextPane;
- import javax.swing.text.BadLocationException;
- import javax.swing.text.Document;
- import javax.swing.text.SimpleAttributeSet;
- import javax.swing.text.StyleConstants;
- public class JTextPaneExample {
- public static void main(String args[]) throws BadLocationException {
- JFrame frame = new JFrame("JTextPane Example");
- frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
- Container cp = frame.getContentPane();
- JTextPane pane = new JTextPane();
- SimpleAttributeSet attributeSet = new SimpleAttributeSet();
- StyleConstants.setBold(attributeSet, true);
-
-
- pane.setCharacterAttributes(attributeSet, true);
- pane.setText("Welcome");
-
- attributeSet = new SimpleAttributeSet();
- StyleConstants.setItalic(attributeSet, true);
- StyleConstants.setForeground(attributeSet, Color.red);
- StyleConstants.setBackground(attributeSet, Color.blue);
-
- Document doc = pane.getStyledDocument();
- doc.insertString(doc.getLength(), "To Java ", attributeSet);
-
- attributeSet = new SimpleAttributeSet();
- doc.insertString(doc.getLength(), "World", attributeSet);
-
- JScrollPane scrollPane = new JScrollPane(pane);
- cp.add(scrollPane, BorderLayout.CENTER);
-
- frame.setSize(400, 300);
- frame.setVisible(true);
- }
- }
Output
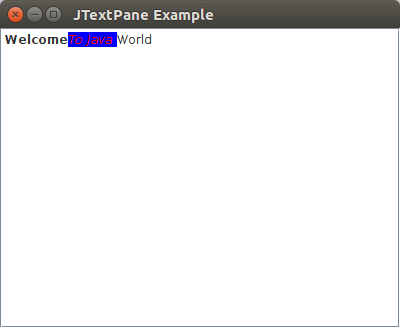
Java JRootPane
JRootPane is a lightweight container used behind the scenes by JFrame, JDialog, JWindow, JApplet, and JInternalFrame.
Nested Classes
Modifier and Type | Class | Description |
---|
protected class | JRootPane.AccessibleJRootPane | This class implements accessibility support for the JRootPane class. |
protected class | JRootPane.RootLayout | A custom layout manager that is responsible for the layout of layeredPane, glassPane, and menuBar. |
Fields
Modifier and Type | Field | Description |
---|
static int | COLOR_CHOOSER_DIALOG | Constant used for the windowDecorationStyle property. |
protected JButton | contentPane | The content pane. |
protected Container | defaultButton | The button that gets activated when the pane has the focus and a UI-specific action like pressing the Enter key occurs. |
protected JMenuBar | menuBar | The menu bar. |
protected Component | glassPane | The glass pane that overlays the menu bar and content pane, so it can intercept mouse movements and such. |
static int | ERROR_DIALOG | Constant used for the windowDecorationStyle property. |
Constructor
Constructor | Description |
---|
JRootPane() | Creates a JRootPane, setting up its glassPane, layeredPane, and contentPane. |
Useful Methods
Modifier and Type | Method | Description |
---|
protected void | addImpl(Component comp, Object constraints, int index) | Overridden to enforce the position of the glass component as the zero child. |
void | addNotify() | Notifies this component that it now has a parent component. |
protected Container | createContentPane() | It is called by the constructor methods to create the default contentPane. |
protected Component | createGlassPane() | It called by the constructor methods to create the default glassPane. |
AccessibleContext | getAccessibleContext() | It gets the AccessibleContext associated with this JRootPane. |
JButton | getDefaultButton() | It returns the value of the defaultButton property. |
void | setContentPane(Container content) | It sets the content pane -- the container that holds the components parented by the root pane. |
void | setDefaultButton(JButton defaultButton) | It sets the defaultButton property, which determines the current default button for this JRootPane. |
void | setJMenuBar(JMenuBar menu) | It adds or changes the menu bar used in the layered pane. |
JRootPane Example
- import javax.swing.JButton;
- import javax.swing.JFrame;
- import javax.swing.JMenu;
- import javax.swing.JMenuBar;
- import javax.swing.JRootPane;
-
- public class JRootPaneExample {
- public static void main(String[] args) {
- JFrame f = new JFrame();
- f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
- JRootPane root = f.getRootPane();
-
-
- JMenuBar bar = new JMenuBar();
- JMenu menu = new JMenu("File");
- bar.add(menu);
- menu.add("Open");
- menu.add("Close");
- root.setJMenuBar(bar);
-
-
- root.getContentPane().add(new JButton("Press Me"));
-
-
- f.pack();
- f.setVisible(true);
- }
- }
Output
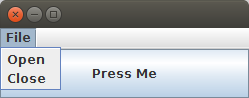
Anurag Rana
Educator CSE/IT
Comments
Post a Comment