C++ Arrays
Like other programming languages, array in C++ is a group of similar types of elements that have contiguous memory location.
In C++ std::array is a container that encapsulates fixed size arrays. In C++, array index starts from 0. We can store only fixed set of elements in C++ array.
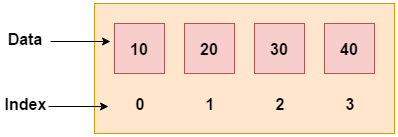
Advantages of C++ Array
- Code Optimization (less code)
- Random Access
- Easy to traverse data
- Easy to manipulate data
- Easy to sort data etc.
Disadvantages of C++ Array
- Fixed size
C++ Array Types
There are 2 types of arrays in C++ programming:
- Single Dimensional Array
- Multidimensional Array
C++ Single Dimensional Array
Let's see a simple example of C++ array, where we are going to create, initialize and traverse array.
Output:/p>
10
0
20
0
30
C++ Array Example: Traversal using foreach loop
We can also traverse the array elements using foreach loop. It returns array element one by one.
Output:
10
20
30
40
50
C++ Passing Array to Function
In C++, to reuse the array logic, we can create function. To pass array to function in C++, we need to provide only array name.
C++ Passing Array to Function Example: print array elements
Let's see an example of C++ function which prints the array elements.
Output:
Printing array elements:
10
20
30
40
50
Printing array elements:
5
15
25
35
45
C++ Passing Array to Function Example: Print minimum number
Let's see an example of C++ array which prints minimum number in an array using function.
Output:
Minimum element is: 10
Minimum element is: 5
C++ Passing Array to Function Example: Print maximum number
Let's see an example of C++ array which prints maximum number in an array using function.
Output:
Maximum element is: 54
Maximum element is: 67
C++ Multidimensional Arrays
The multidimensional array is also known as rectangular arrays in C++. It can be two dimensional or three dimensional. The data is stored in tabular form (row ∗ column) which is also known as matrix.
C++ Multidimensional Array Example
Let's see a simple example of multidimensional array in C++ which declares, initializes and traverse two dimensional arrays.
Output:
5 10 0
0 15 20
30 0 10
C++ Multidimensional Array Example: Declaration and initialization at same time
Let's see a simple example of multidimensional array which initializes array at the time of declaration.
Output:"
2 5 5
4 0 3
9 1 8
Comments
Post a Comment